Streamlining YouTube Integration: Icon Techniques & Best Practices for Optimised Video Loading
When it comes to enhancing engagement on articles, having video content available can be highly effective. One challenge with having video content available on your site is making people aware that it exists! Traditionally a solution for this is to overlay a play button on the image, indicating video availability on clickthrough. However, traditional methods of loading play button overlays are often clunky (huge amounts of CSS and markup) and/or inefficient (generating thumbnails with play buttons saved on them).
Once users come to the articles with videos on them, embedding YouTube videos directly on web pages often leads to Google's PageSpeed tool complaining about inefficiencies and slow page loads. In this article, we'll explore some solutions for integrating YouTube videos and icons while maintaining good web performance.
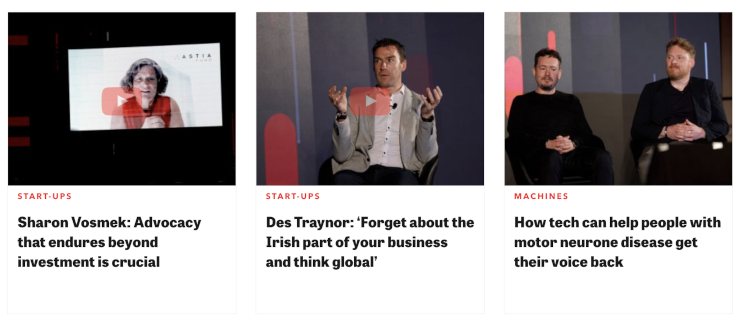
Option 1: SVG Icon Approach
Using Scalable Vector Graphics (SVG) for icons offers a performant solution. SVG icons are resolution-independent, providing sharp and crisp visuals on any screen. Additionally, they are lightweight and can be easily styled with CSS. Let's take a look at a sample code snippet for an SVG YouTube icon overlay:
First, the HTML:
<div class="image-container">
<img src="https://i3.ytimg.com/vi/4u34udxw6l8/hqdefault.jpg"
alt="Exciting video">
<div class="overlay-container">
<div class="play-button"></div>
</div>
</div>
Then the CSS:
.image-container {
position: relative;
}
.overlay-container {
/* Take up full size of the image */
position: absolute;
top: 0;
left: 0;
height: 100%;
width: 100%;
/* Ensure our icon is centered */
display: flex;
align-items: center;
justify-content: center;
opacity: 0.4;
background: black;
}
.overlay-container:hover {
cursor: pointer;
opacity: 1;
background: transparent;
}
.overlay-container > .play-button {
width: 5rem;
height: calc(5rem * 0.7); /* Maintain aspect ratio */
cursor: pointer;
background-color: transparent;
background-image: url('data:image/svg+xml;utf8,<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 68 48"><path d="M66.52 7.74c-.78-2.93-2.49-5.41-5.42-6.19C55.79.13 34 0 34 0S12.21.13 6.9 1.55c-2.93.78-4.63 3.26-5.42 6.19C.06 13.05 0 24 0 24s.06 10.95 1.48 16.26c.78 2.93 2.49 5.41 5.42 6.19C12.21 47.87 34 48 34 48s21.79-.13 27.1-1.55c2.93-.78 4.64-3.26 5.42-6.19C67.94 34.95 68 24 68 24s-.06-10.95-1.48-16.26z" fill="red"/><path d="M45 24 27 14v20" fill="white"/></svg>');
transition: filter .1s cubic-bezier(0, 0, 0.2, 1);
border: none;
}
The code for the SVG icon is taken from Paul Irish's excellent Lite YouTube facade - more on that later!
The steps we've taken here are:
- Create containers for the image and overlay
- Create an overlay container that takes up the full size of the image
- Create a play button icon that is centered within the overlay container. Inlining the SVG here means we don't need an additional network request
- Add opacity to the overlay CSSContainerRule, so the icon doesn't appear too brightly
- Add a hover effect to the overlay container to remove the opacity, better highlighting the play button
Full Colour Font Awesome YouTube Icons
If your website is already loading the Font Awesome icon set, you can take advantage of the YouTube icon already included in this set.
<i class="fab fa-youtube" style="color: red;"></i>
The fab
class here tells Font Awesome we want to use one of the brand icons, with fa-youtube
specifying the logo we want. Setting the colour of the icon to red leaves us with this:
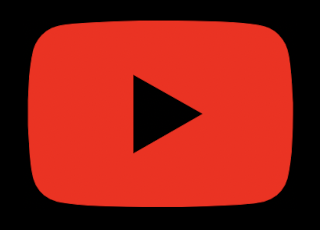
One thing to notice here is that the icon is "hollow", i.e. the play button will inherit whatever the colour of our background is. If we're going to have this image as an overlay on our article thumbnails, we might get some odd colour clashes there! Ideally we want to to copy the full YouTube logo, with the white inset. But how to do that with Font Awesome? Enter stacks!
Stacks are a concept in Font Awesome where we can layer icons on top of one another. Consider this example:
<span class="fa-stack fa-2x">
<i class="fas fa-circle fa-stack-1x" style="color: white;"></i>
<i class="fab fa-youtube fa-stack-2x" style="color: red;"></i>
</span>
- The
fa-stack
class on the parentspan
sets us up for a stacked set of icons.fa-2x
here is going to size the icon to 2x normal size, which better reflects the typical size of a YouTube play button on embeds. fas fa-circle fa-stack-1x
-fas fa-circle
means we're going to create a circle from the "solid" Font Awesome icons. Herefa-stack-1x
means this icon will have the same size as the default size for the stack -fa-2x
in our case. We're setting the colour of this icon towhite
.fab fa-youtube fa-stack-2x
-fab
means we're going to use a brand icon, withfa-youtube
specifying the YouTube play icon. Thefa-stack-2x
setting is key here - this means that this icon will be 2x the base size for our stack group. With the circle being 1x size, it means that the circle will be smaller, will appear in the middle of the icon, and will have no edges showing around the edge of the play icon.
The above means that, even on a black background, our icon will show as a full colour YouTube icon - red with a white fill.
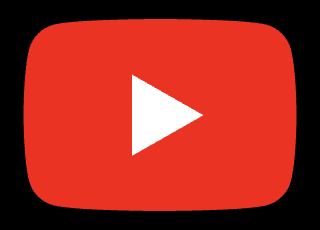
Efficient YouTube embed loading
Now that we've got our play icons indicating video availability, our users will come rushing to the articles on which we have videos. Our next challenge? Ensuring that those pages load as quickly and as efficiently as possible.
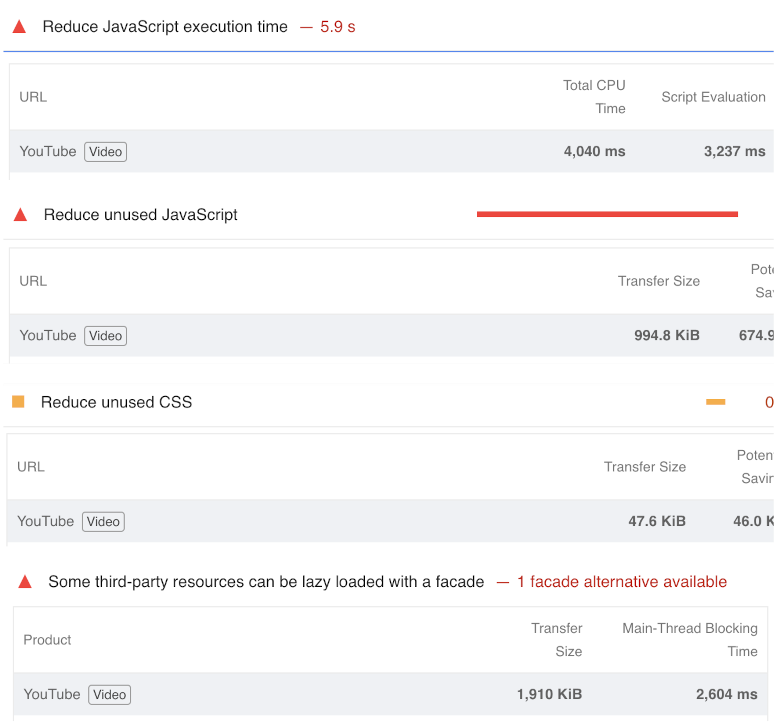
When embedding a YouTube video in a page, Google's Page Speed Insights tool often complains about a number of different factors which impact page load speed. Some of these factors are shown above, and will be familiar to anyone who has worked with YouTube embeds on a performance-sensitive site in the past!
One way to avoid the impact of a YouTube embed on a page is to not load the embed on initial page load, but to only do so when needed. At a high level, we would do something like:
- Have a placeholder image with play button
- When clicked, use javascript to swap the image for a dynamically-inserted YouTube embed
- Play!
This approach means that we avoid taking the penalty of a YouTube embed on each page, and only load the embed when the user has indicated they want to watch the video. This is a good approach, though does rely on the swap stage happening quickly enough to be barely perceptible to the end user. It also requires us to write a bunch of javascript to handle the swap. How can we simplify the development required, while also ensuring that the swap happens as quickly as possible? Enter Lite YouTube Embed!
Lite YouTube Embed is a web component created by Paul Irish, which allows us to embed YouTube videos in a page without the performance penalty of a full embed. In it's own words, it Renders faster than a sneeze
! It works by creating a placeholder image, then swapping in the YouTube embed when the user clicks on the placeholder. It also uses clever DNS prefetching and preconnecting on hover to ensure that the swap happens as quickly as possible.
// On hover (or tap), warm up the TCP connections we're (likely) about to use.
this.addEventListener('pointerover', LiteYTEmbed.warmConnections, {once: true});
Integrating Lite YouTube Embed is surprisingly simple! First, install the assets into your project, either via npm, or via direct download from the project repo.
The styles and js files from the project must be copied into your application. Then, to embed a YouTube video, we simply add the following markup:
<lite-youtube videoid="4u34udxw6l8"></lite-youtube>
By default, the component will show the thumbnail from the video as the placeholder image. However, we can also specify our own placeholder image, which is what we'll do here:
<lite-youtube videoid="4u34udxw6l8"
style="background-image: url('/i/diego-iconic.webp');"></lite-youtube>
This will create a placeholder image with a play button, which will swap in the YouTube embed when clicked. The placeholder image is created by inlining the SVG icon we saw earlier, and is styled with CSS to match the size of the YouTube embed. This means that the swap is almost imperceptible to the end user, and we don't need to write any javascript to handle the swap.
The impact on the resources required on page load is significant. The graphic below shows the typical amount of resources required by a standard YouTube embed, compared to the Lite YouTube Embed. The Lite YouTube Embed is a fraction of the size, and requires a fraction of the resources to load.
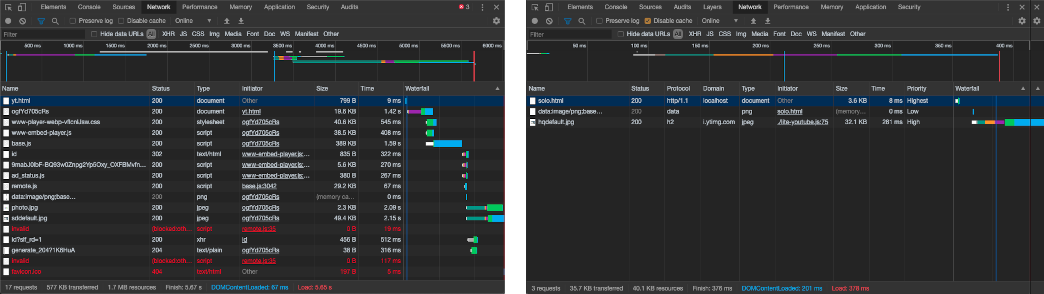
Potential SEO Impact of removing video embeds
One thing to note when removing embeds from your page is the potential SEO impact. With youtube videos embedded into a page, Google's search crawlers can recognise these videos, and potentially include your article in the video search results pages. For some publishers, this can be a significant driver of traffic. Removing the embeds from your page markup prevents Google's crawlers from automatically recognising the embeds.
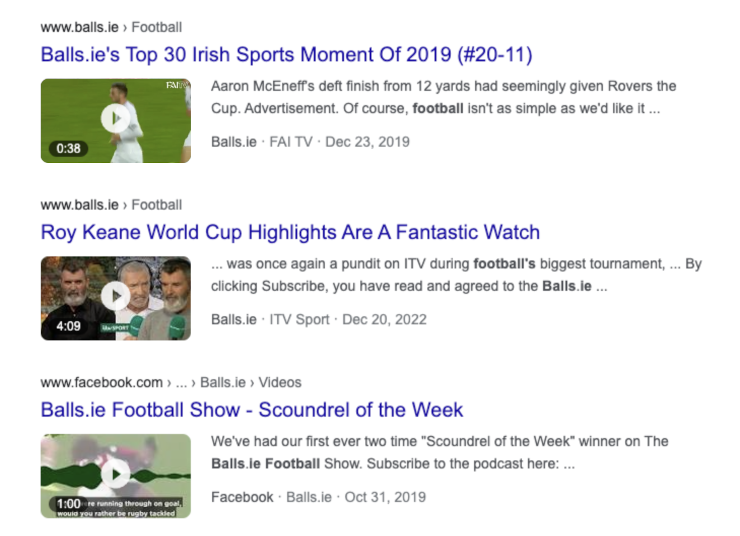
The solution to this is to ensure that you are using structured data on the page, specifically the VideoObject
schema.
<script type="application/ld+json">
{
"@context": "https://schema.org",
"@type": "VideoObject",
"name": "Introducing the self-driving bicycle in the Netherlands",
"description": "This spring, Google is introducing the [...] that will have great impact in their home country.",
"thumbnailUrl": [
"https://example.com/photos/1x1/photo.jpg",
"https://example.com/photos/4x3/photo.jpg",
"https://example.com/photos/16x9/photo.jpg"
],
"uploadDate": "2016-03-31T08:00:00+08:00",
"duration": "PT1M54S",
"contentUrl": "https://www.example.com/video/123/file.mp4",
"embedUrl": "https://www.example.com/embed/123",
"interactionStatistic": {
"@type": "InteractionCounter",
"interactionType": { "@type": "WatchAction" },
"userInteractionCount": 5647018
},
"regionsAllowed": "US,NL"
}
</script>
This structured data object will ensure that Google can still recognise the link between your article content and your video. More information and examples of this structured data object can be found here.
Summary
Integrating YouTube videos and icons on web pages doesn't have to compromise performance. By using SVG icons or cleverly managing the YouTube embeds, you can optimize your website's loading speed and provide a seamless user experience. These best practices can help to improve engagement, whilst continuing to deliver a fast and responsive experience for your visitors!
TL;DR
- Use video availability indicators to show video content exists
- SVG icons are a lightweight way to create brand-specific indicators
- If you're already using Font Awesome, stacks are a great way to create layered, full colour YouTube icons
- Use Lite YouTube Embed to embed YouTube videos on your page without incurring a performance penalty
- Make sure you're using structured data to let search engines discover the link between your article and video
IPC Munich 2025
In October 2025, I'll be speaking at the International PHP Conference in Munich. I'll be talking about Modern PHP Features You’re Probably Not Using (But Should Be). Expect real-world examples, practical takeaways, and a deep dive into cleaning your code while making your life easier!
Get your ticket now and I'll see you there!